- Published on, Time to read
- 🕒 3 min read
Optimizely / Episerver: How to create asset folder programmatically?
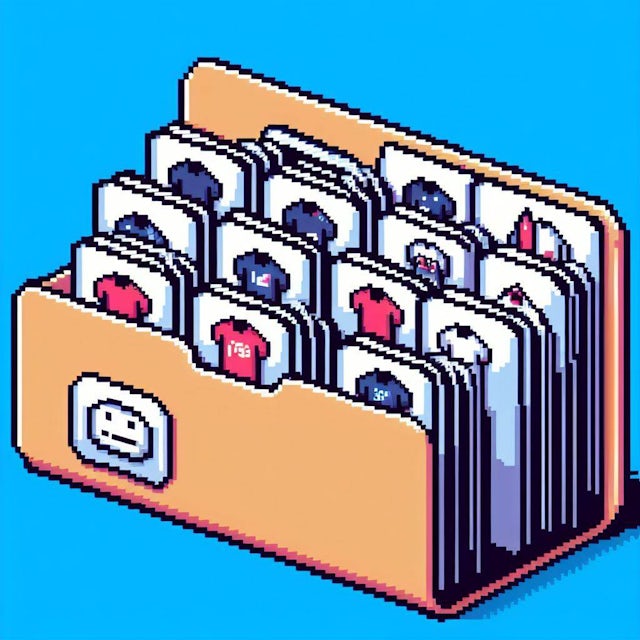
Table of contents:
- Create an asset folder for a specific page
- Create an asset sub-folder for all pages
- In practice
- Loading media from the asset folder
- Example of usage
- Links
Introduction
In Optimizely CMS, asset folders are used to organize media files. According to the docs:
Assets such as blocks and media files, are available from edit view in the assets pane in Optimizely Content Management System (CMS) and Optimizely Customized Commerce. Assets can also be product items in the product catalog in Optimizely Customized Commerce. A media type can be for example an image, a video, or a document in a defined file format.NoteLike other content types, media types must first be defined in the content model, to use the built-in functionality for assets. If a media type is not defined on the website, file upload or drag and drop will not work.
Assets might be defined for the global scope or for a specific page. For example, the following screenshot shows the global assets folder—"For All Sites"—while the specific page assets folder is shown as "For This Page".
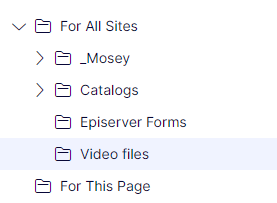
You can create an asset folder in the CMS UI, but you can also create it programmatically. In this blog post, I will present how to create an asset folder programmatically.
Create an asset folder for a specific page
Creation of an asset folder for a specific page is pretty straightforward. You need to get the ContentAssetHelper
(the class does not implement any of interface) and call the GetOrCreateAssetFolder
.
For example, the following code snippet creates an asset folder for the start page:
var startPage = ContentReference.StartPage;
_contentAssetHelper.GetOrCreateAssetFolder(startPage);
Create an asset sub-folder for all pages
In order to create an asset sub-folder for all pages, you need to:
- get children of
ContentFolder
from the Global Assets folder - get default instance of the
ContentFolder
type - save the above
The following code snippet creates an asset folder for all pages in the CMS.
public ContentReference GetOrCreateGlobalAssetFolder(string name)
{
var assetsReference = SystemDefinition.Current.GlobalAssetsRoot;
var contentFolders = _contentRepository.GetChildren<ContentFolder>(assetsReference)
.ToList();
var dir = contentFolders.FirstOrDefault(x => x.Name == name);
if (dir != null && !ContentReference.IsNullOrEmpty(dir.ContentLink))
{
return dir.ContentLink;
}
var newDirectory = _contentRepository.GetDefault<ContentFolder>(assetsReference);
newDirectory.Name = name;
var assetFolder = _contentRepository.Save(newDirectory,
SaveAction.Publish, AccessLevel.NoAccess);
return assetFolder;
}
In practice
When invoking the code for creating an asset folder for all pages:
_contentAssetService.GetOrCreateGlobalAssetFolder("Video files");
...then the new folder is created under "For All Sites" (GlobalAssetsRoot
) directory:
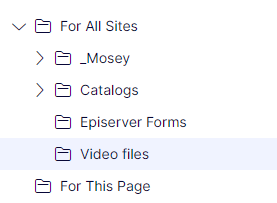
Loading media from the asset folder
In order to traverse the asset folder, you might use the GetChildren
method from the ContentRepository
:
var assetFolder = _assetService.GetOrCreateGlobalAssetFolder("your directory name");
var media = _contentRepository.GetChildren<YourContentType>(assetFolder)
Example of usage
The example of usage can be found on my fork of the Foundation project at the GitHub:
Links
- Assets and media (CMS Dev Guide)